Introduction:
In this tutorial you will learn how to interface HX711 loadcell amplifier module with ESP8266 NodeMCU board and getting output on i2c OLED display. Also, we will learn how to add zero push button function to adjust scale offset.
Loadcell:
Load cell is a sensor which converts mechanical force into electronic signal. Where the mechanical force can be tension, pressure, compression or torque. There are many types of load cells are available in the market which can be used as per required application. In this example we are going to use strain gauge load cell for converting mechanical force into electrical signal.
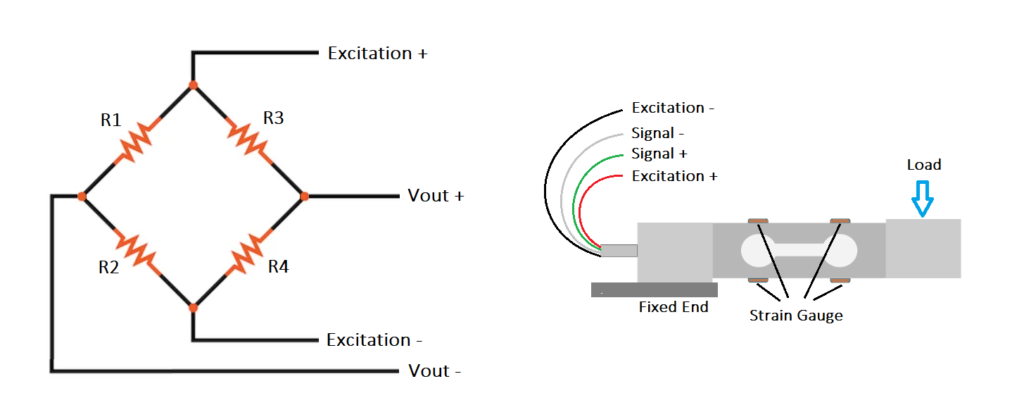
Loadcell consist of several resistive strain gauge sensor elements which changes its resistance when the load is applied and gives output in milli volts when input or excitation voltage is applied to it.
This milli volt output is then amplified to voltage signal to make it compatible with controllers to read and convert to load units.
Things You Will Need:
- ESP8266 NodeMCU
- HX711 Amplifier Board
- 10kg Loadcell
- i2c OLED Display
- Connecting Cables
Circuit Diagram:
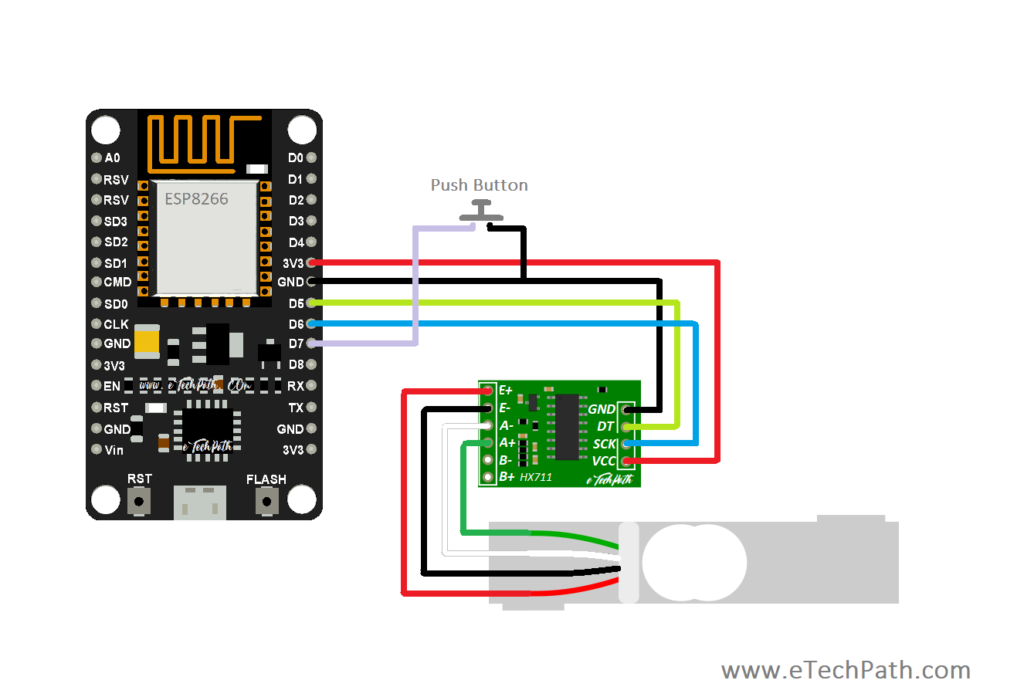
Code:
Example 1: ln this example we will use Arduino IDE serial monitor window to get output values from the loadcell.
/**
*
* Interfacing 10kg loadcell and HX711 amplifier board with ESP8266 NodeMCU
* Author: Pranay Sawarkar
* Website: www.eTechPath.com
* Link: https://blog.etechpath.com/diy-weighing-scale-using-hx711-oled-i2c-display-and-esp8266-nodemcu-with-zero-calibration-function/
*
*
**/
#include <Arduino.h>
#include "HX711.h"
// HX711 circuit wiring
const int Dout_Pin = 14;
const int SCK_Pin = 12;
const int pb1 = 13; //push button input
int tarepb = 0;
int newread = 0;
#define CalFactor 235.5 //enter your calibration factor here
HX711 scale;
void setup() {
Serial.begin(115200);
scale.begin(Dout_Pin, SCK_Pin);
pinMode(pb1, INPUT_PULLUP);
Serial.println("Initialization...");
scale.set_scale(CalFactor);
scale.tare();
delay (200);
Serial.println("Ready");
delay (100);
}
void loop() {
tarepb = digitalRead(pb1);
delay(10);
if (tarepb == LOW)
{
scale.tare();
Serial.println("TareDONE");
}
else
{
newread = scale.get_units(5);
Serial.println("Weight: ");
Serial.println(newread);
delay(10);
}
}
Circuit Diagram:
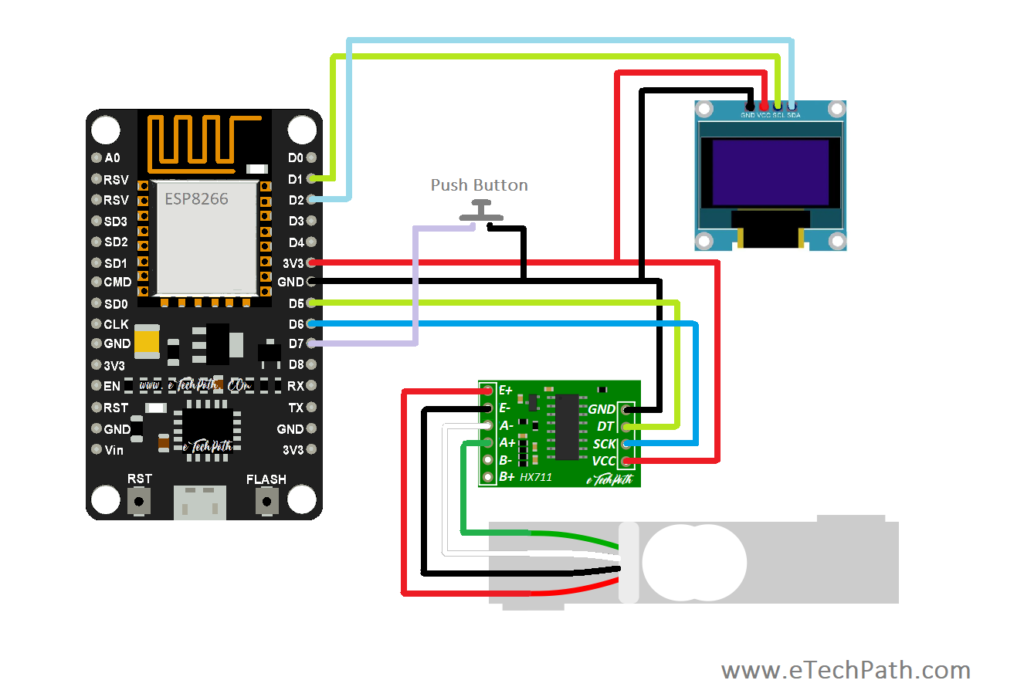
Example 2: In this example we will interface oled display with the existing circuit and print loadcell output values on display.
/**
*
* DIY Weighing Scale using HX711, OLED i2c display and ESP8266 NodeMCU with Zero Calibration Function
* Author: Pranay Sawarkar
* Website: www.eTechPath.com
* Link: https://blog.etechpath.com/diy-weighing-scale-using-hx711-oled-i2c-display-and-esp8266-nodemcu-with-zero-calibration-function/
*
*
**/
#include <Arduino.h>
#include <U8g2lib.h>
#include "HX711.h"
#ifdef U8X8_HAVE_HW_SPI
#include <SPI.h>
#endif
#ifdef U8X8_HAVE_HW_I2C
#include <Wire.h>
#endif
//select your oled display size
U8G2_SSD1306_128X32_UNIVISION_F_HW_I2C u8g2(U8G2_R0, /* reset=*/ U8X8_PIN_NONE);
//U8G2_SSD1306_128X64_NONAME_F_HW_I2C u8g2(U8G2_R0, /* reset=*/ U8X8_PIN_NONE);
// HX711 circuit wiring
const int Dout_Pin = 14;
const int SCK_Pin = 12;
const int pb1 = 13;
//const int pb2 = 5;
int tarepb = 0;
int newread = 0;
#define CalFactor 235.5
//235.5
HX711 scale;
void setup() {
Serial.begin(115200);
u8g2.begin();
scale.begin(Dout_Pin, SCK_Pin);
pinMode(pb1, INPUT_PULLUP);
u8g2.clearBuffer();
u8g2.setFont(u8g2_font_6x10_tf);
u8g2.drawStr(0, 20, "Initializing...");
u8g2.sendBuffer();
scale.set_scale(CalFactor);
scale.tare();
delay (500);
u8g2.clearBuffer();
u8g2.drawStr(0, 20, "Ready");
u8g2.sendBuffer();
delay (100);
}
void loop() {
tarepb = digitalRead(pb1);
delay(10);
if (tarepb == LOW)
{
scale.tare();
u8g2.clearBuffer();
u8g2.drawStr(0, 20, "Tare Done");
Serial.println("TareDONE");
u8g2.sendBuffer();
}
else
{
newread = scale.get_units(5);
Serial.println(newread);
delay(10);
u8g2.clearBuffer();
u8g2.setFont(u8g2_font_6x10_tf);
u8g2.drawStr(0, 20, "Weight: ");
u8g2.setCursor(45, 20);
u8g2.print(newread);
u8g2.sendBuffer();
delay(10);
}
}
Prototype:
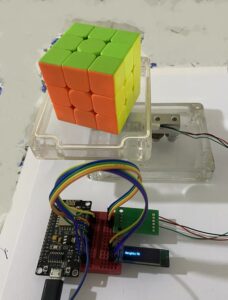
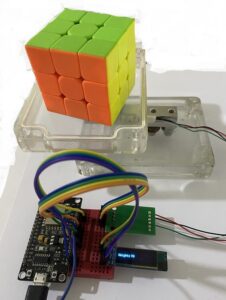