In this tutorial, you will learn how to interface MAX6675 thermocouple amplifier module with node MCU ESP8266 and view sensor reading on esp local webserver without using any router.
Table of Contents:
- Types of temperature sensors
- MAX6675 HW-550 Module
- Interfacing MAX6675 with ESP
- Installing libraries
- Examples to read temperature from MAX6675
Types of temperature sensors.
There are four measure types of temperature sensors that are commonly used in the industry: RTD, Thermocouples, Thermistor and semiconductor based IC’s. From these four types, we will talk about the Thermocouple temperature sensors in this tutorial.
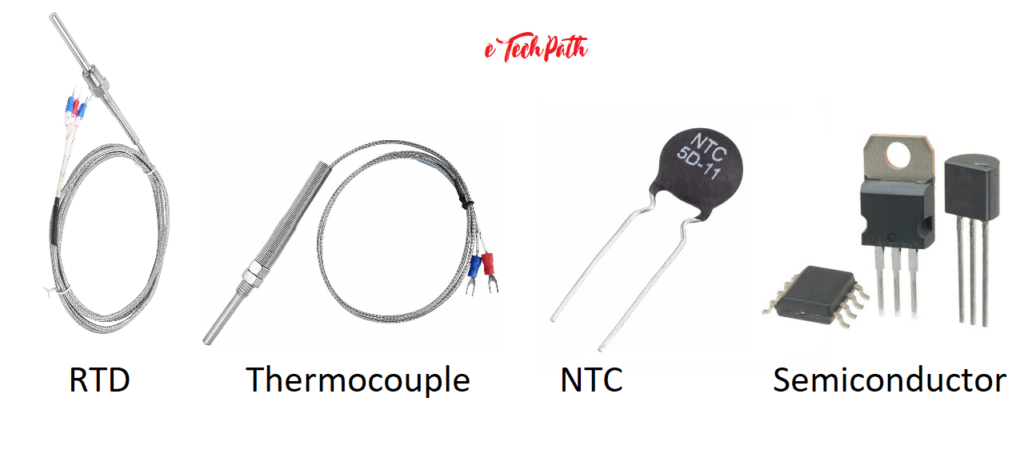
Thermocouple is a temperature sensor which contains two wires and gives output in millivoltage with respect to junction temperature. This temperature sensor wires also has fixed polarity, So you can not reverse it.
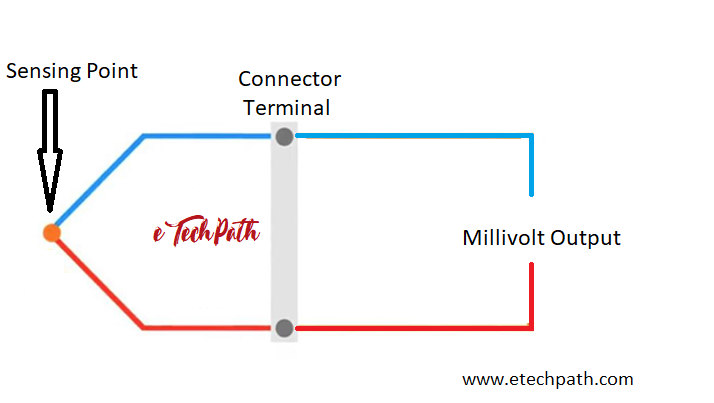
Sensor element of thermocouple is made up of two different types of metals which is joint together at one point. When this point gets heated or cooled, a voltage is created that can be use as reference for temperature calculation.
Max6675 Module:
MAX6675 is k-type thermocouple to digital converter which provides output in SPI serial interface with 12-bit resolution. MAX6675 can measure temperature range from 0°C to 1024°C with the accuracy of 0.25°C
- Supply Voltage: 3.0V to 6.0V DC
- Current: 50mA
- Operating temperature : -20°C to +80°C
Schematic Diagram:
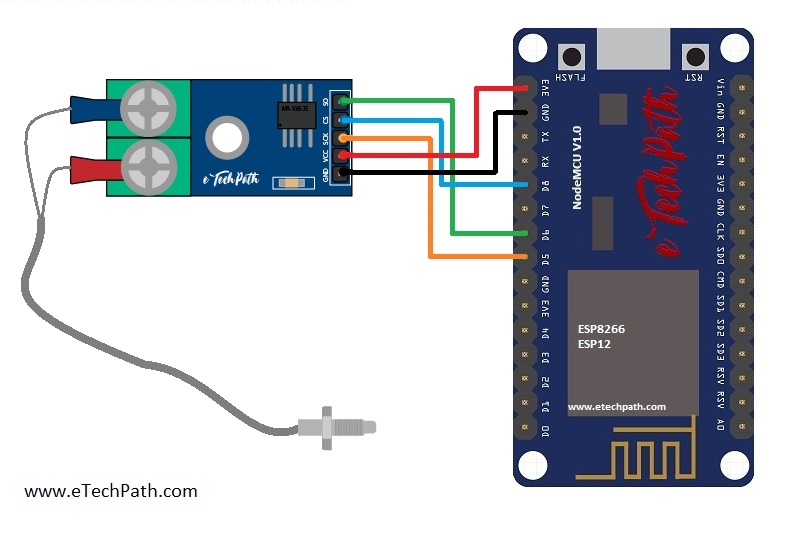
Installing Arduino Libraries:
- First you will have to install ESP8266 board manager into Arduino IDE, if you have not installed it yet. Follow this simple tutorial
- Install MAX6675 library in Arduino IDE. GitHub link to download library. (ZIP)
- Install ESPAsyncWebServer library in Arduino IDE. GitHub link to download library. (ZIP)
Examples:
Interfacing MAX6675 with ESP8266 and monitoring temperature in serial monitoring.
#include <Arduino.h>
#include <ESP8266WiFi.h>
#include "max6675.h"
int thermoDO = 12;
int thermoCS = 15;
int thermoCLK = 14;
MAX6675 thermocouple(thermoCLK, thermoCS, thermoDO);
void setup() {
Serial.begin(115200);
Serial.println("MAX6675 test");
// Stabilisation delay for MAX6675 chip
delay(500);
}
void loop() {
Serial.print("C = ");
Serial.println(thermocouple.readCelsius());
Serial.print("F = ");
Serial.println(thermocouple.readFahrenheit());
// There should be at-least 250ms delay between reeds from MAX 6675
delay(1500);
}
Interfacing MAX6675 with ESP8266 and monitoring temperature in ESP local webserver.
#include <Arduino.h>
#include <ESP8266WiFi.h>
#include <Hash.h>
#include <ESPAsyncTCP.h>
#include <ESPAsyncWebServer.h>
#include "max6675.h"
const char* ssid = "MAX6675-Server";
const char* password = "12341234";
int thermoDO = 12;
int thermoCS = 15;
int thermoCLK = 14;
MAX6675 thermocouple(thermoCLK, thermoCS, thermoDO);
float t = 0.0;
float f = 0.0;
AsyncWebServer server(80);
unsigned long previousMillis = 0; //will store last time temp was updated
const long interval = 1000;
const char index_html[] PROGMEM = R"rawliteral(
<!DOCTYPE HTML><html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1">
<style>
html {
font-family: Arial;
display: inline-block;
margin: 0px auto;
text-align: center;
}
h2 { font-size: 3.0rem; }
p { font-size: 3.0rem; }
.units { font-size: 1.2rem; }
.temp-labels{
font-size: 1.5rem;
vertical-align:middle;
padding-bottom: 10px;
}
</style>
</head>
<body>
<h2>Max6675 Thermocouple Server</h2>
<h3>www.eTechPath.com</h3>
<p>
<span class="temp-labels">Temperature</span>
</p>
<p>
<span id="temperature">%TEMPERATURE%</span>
<sup class="units">°C</sup>
</p>
<p>
<span id="fahrenheit">%FAHRENHEIT%</span>
<sup class="units">°F</sup>
</p>
</body>
<script>
setInterval(function ( ) {
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
document.getElementById("temperature").innerHTML = this.responseText;
}
};
xhttp.open("GET", "/temperature", true);
xhttp.send();
}, 1000 ) ;
setInterval(function ( ) {
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
document.getElementById("fahrenheit").innerHTML = this.responseText;
}
};
xhttp.open("GET", "/fahrenheit", true);
xhttp.send();
}, 10000 ) ;
</script>
</html>)rawliteral";
// Replaces placeholder with sensor values
String processor(const String& var){
if(var == "TEMPERATURE"){
return String(t);
}
else if(var == "FAHRENHEIT"){
return String(f);
}
return String();
}
void setup(){
Serial.begin(115200);
Serial.print("Setting AP (Access Point)…");
WiFi.softAP(ssid, password);
IPAddress IP = WiFi.softAPIP();
Serial.print("AP IP address: ");
Serial.println(IP);
Serial.println(WiFi.localIP());
// Route for root
server.on("/", HTTP_GET, [](AsyncWebServerRequest *request){
request->send_P(200, "text/html", index_html, processor);
});
server.on("/temperature", HTTP_GET, [](AsyncWebServerRequest *request){
request->send_P(200, "text/plain", String(t).c_str());
});
server.on("/fahrenheit", HTTP_GET, [](AsyncWebServerRequest *request){
request->send_P(200, "text/plain", String(f).c_str());
});
server.begin();
}
void loop()
{
unsigned long currentMillis = millis();
if (currentMillis - previousMillis >= interval)
{
previousMillis = currentMillis;
// Read Celsius
float newT = thermocouple.readCelsius();
if (isnan(newT))
{
Serial.println("Failed to read from Thermocouple Sensor!");
}
else
{
t = newT;
Serial.println(t);
}
// Read Fahrenheit
float newF = thermocouple.readFahrenheit();
if (isnan(newF))
{
Serial.println("Failed to read from Thermocouple Sensor!");
}
else
{
f = newF;
Serial.println(f);
Serial.println(WiFi.softAPIP());
}
}
}
Prototype:
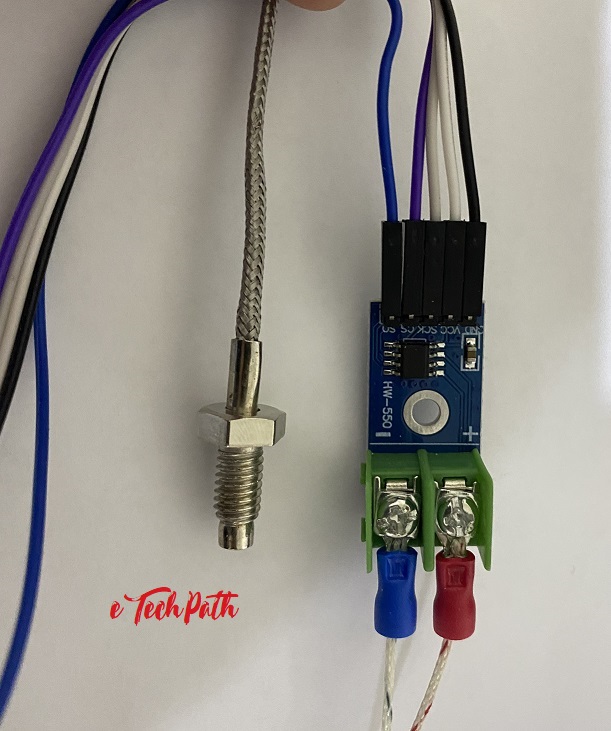
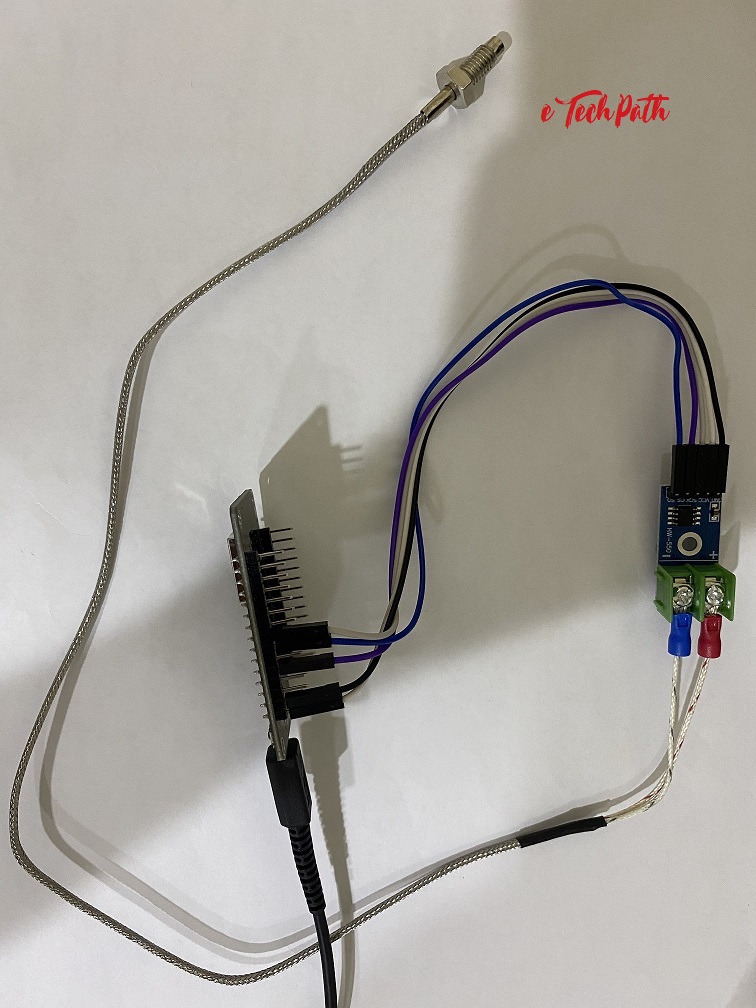
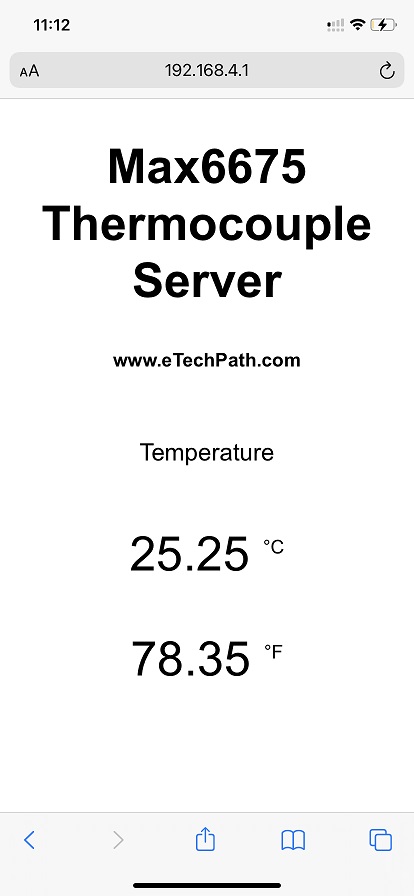
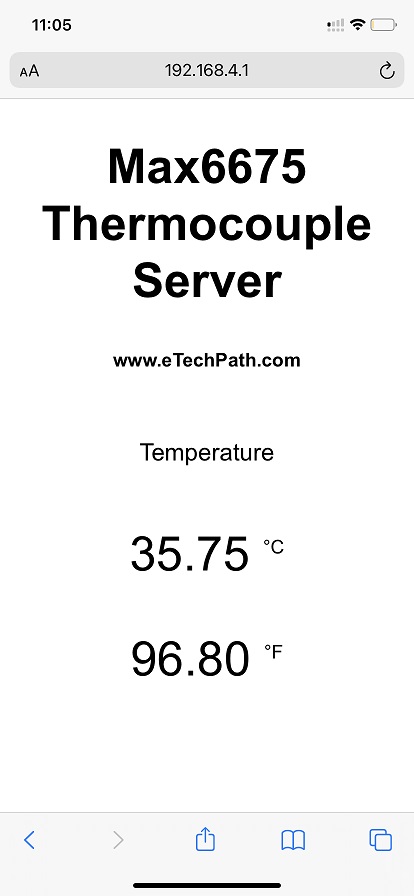